Aurizn evolved from the merger of two leading technology solutions companies, bringing together two decades of experience and a unique set of capabilities to serve the Defence and Enterprise sectors.
Our History
Aurizn is a new brand with a long track record of cutting edge skills and delivery.
Aurizn evolved from the merger of two leading technology organisations, Consilium and elmTEK in 2022.
The two companies had been each serving the Defence sector for more than a decade.
The combination of elmTEK and Consilium’s deep expertise and specialities has enabled Aurizn to bring to market a unique offer, covering Artificial Intelligence, Simulation and Advanced Sensors.
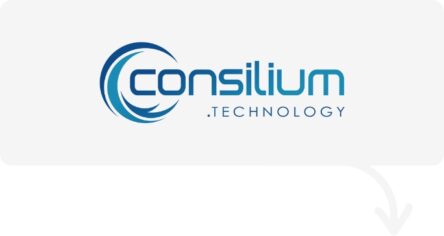
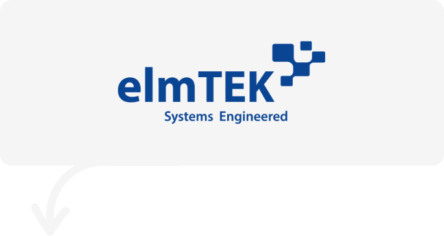